Game Over
When your game ends, you should call Rune.gameOver(options)
to inform Rune that the game ended. As a result, Rune will overlay a standardized game over popup to the user.
This popup contents will vary depending on the options you pass. The main choice is whether the game has winners/losers or assigns each player a score.
Coop Games
If your game has a common goal for all players, you can use everyone
to provide a common result for all players.
// logic.js
Rune.initLogic({
actions: {
myAction: (payload, { game }) => {
Rune.gameOver({ everyone: 300 })
},
},
})
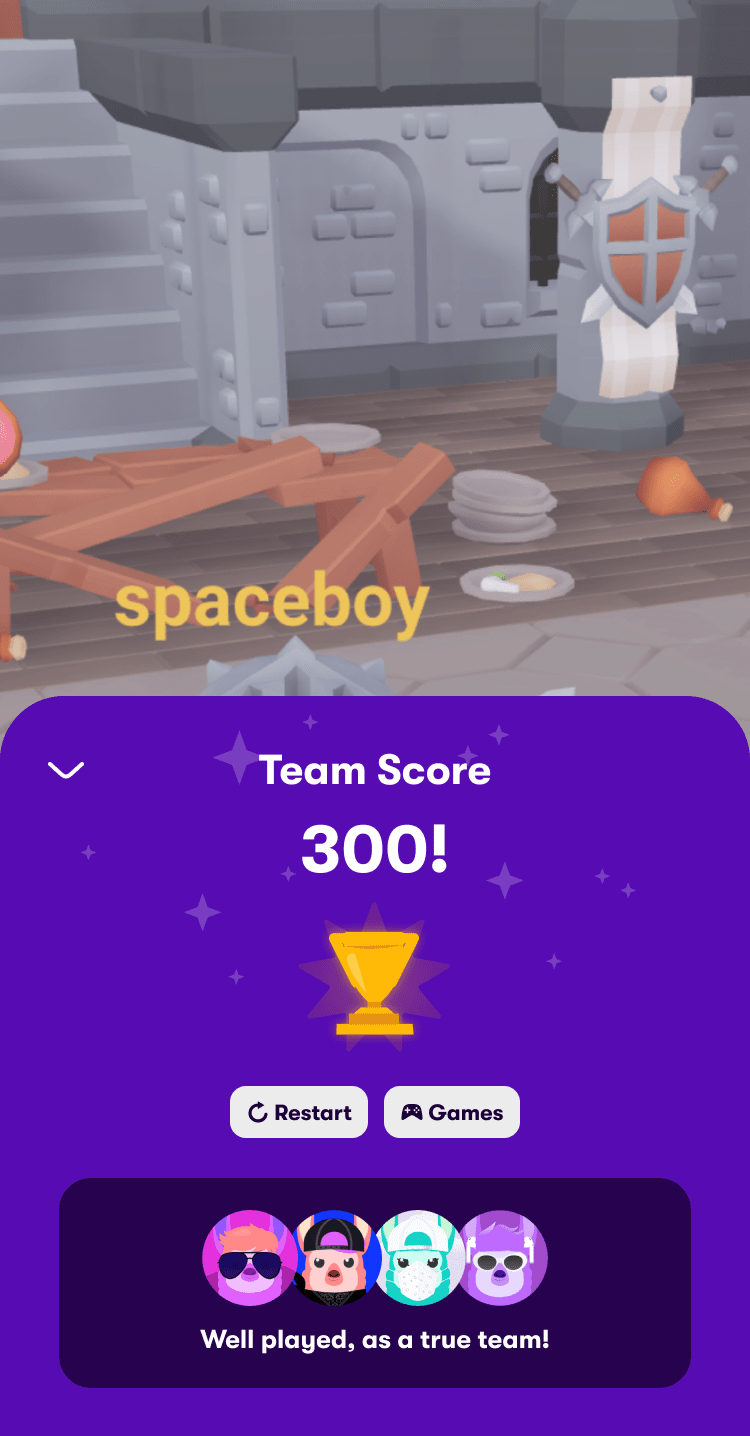
Winners, Losers And Ties
We support all kinds of combinations of winners, losers and ties and the game over popup will change accordingly. You can have a single winner/loser, many winners/losers/ties or have everyone win/lose/tie. The UI will also look different depending on whether the current player is among the winners, losers, ties or is a spectator.
// logic.js
Rune.initLogic({
actions: {
myAction: (payload, { game }) => {
if (isGameOver(game)) {
const winner = getWinner(game)
const loser = getLoser(game)
Rune.gameOver({
players: {
[winner.playerId]: "WON",
[loser.playerId]: "LOST",
},
})
}
},
},
})
Winner | Loser | Tie | Spectator |
---|---|---|---|
![]() | ![]() | ![]() | ![]() |
Player Scores
If your game assigns each player with a score, Rune will show a leaderboard in the game over popup, highlighting the current player. The player with with the highest score wins.
// logic.js
Rune.initLogic({
actions: {
myAction: (payload, { game, allPlayerIds }) => {
if (isGameOver(game)) {
Rune.gameOver({
players: {
[allPlayerIds[0]]: 21981,
[allPlayerIds[1]]: 8911,
[allPlayerIds[2]]: 20109,
[allPlayerIds[3]]: 323,
},
})
}
},
},
})
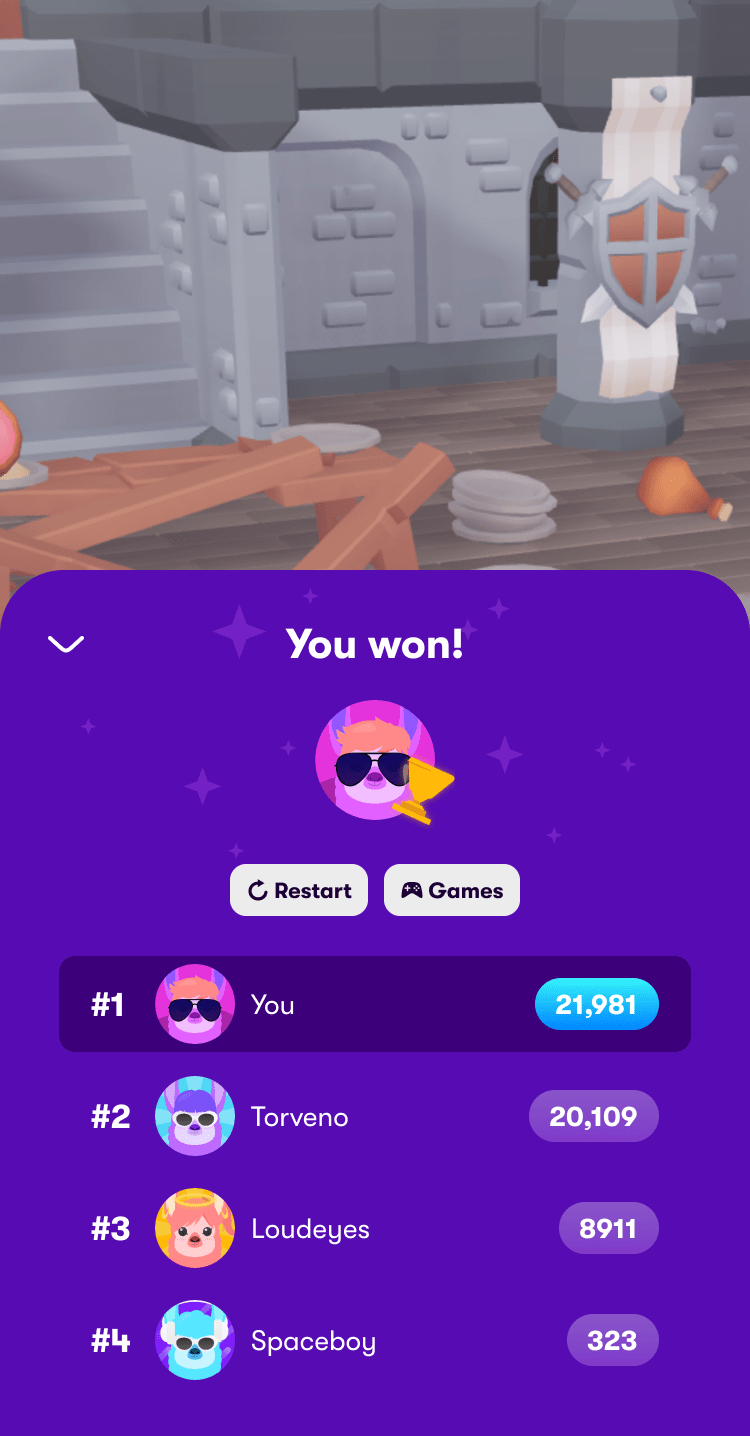
Minimizing Game Over Popup
If you want to build a custom game over screen, you can pass minimizePopUp: true
to Rune.gameOver()
. This will force the popup to initially be minimized.
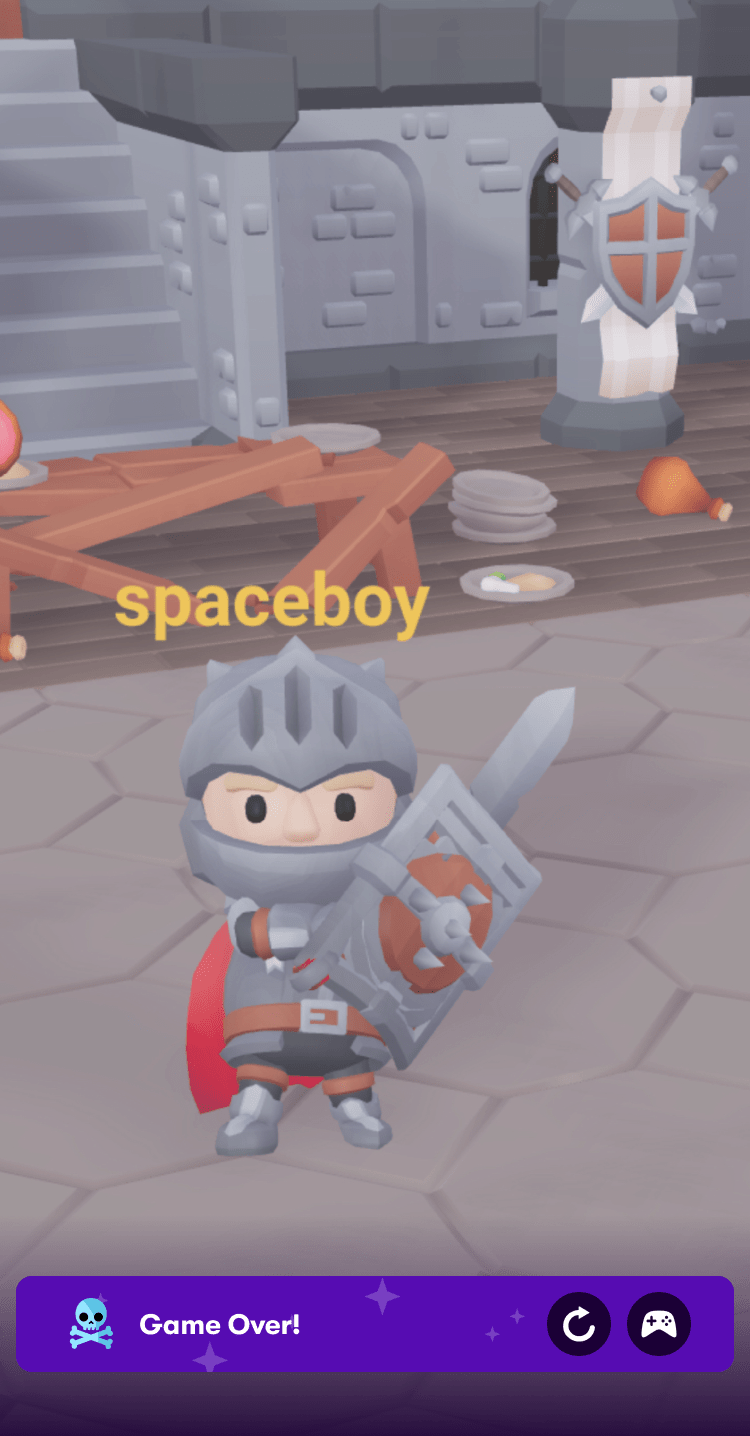
Delaying Game Over Popup
If you pass delayPopUp: true
to Rune.gameOver()
, Rune will not show the game popup immediately. This is useful if you want to e.g. display some animation or just make sure that the players see the final game state before the game over popup is shown. In this case, you should call Rune.showGameOverPopUp()
in your client.js
. If you don't do it, Rune will still show it automatically after a few seconds.